Geldautomat using Java Card
Enthusiasm towards learning Java Card technology has motivated me to develop this application. The application would be very useful for the beginners to study and develop smart card applications. Also, it helps user to understand and develop java card applets. I have published application code on my github repo which I developed for the demonstration.
Two applications have been developed, one is a wallet applet which runs on the smartcart. The applet consists of features like Debit, Credit, Balance, PIN Verification, and Card Un-blocking. The other one is, a host application which runs on the PC and communicates with the applet using APDU (application protocol data units). The applications are developed in Java utilizing java card APIs on NetBeans environment. The communication with the simulator is established on TCP socket port 9025. So, I have programmed to activate the socket to establish communication between applet and host application. Also, I have designed a Swing GUI to set the commands and data for interaction with the host application.
Demo
Now let's study the fundamentals of Java Card technology.
Overview
When a Java Card applet is installed an instance is created and registered with the (Java card runtime environment) JCRE's registry table. The host application sets up the connection to the port 9025 to communicate with the applet. The JCRE selects an applet on the card based on the incoming select commands. Each applet is identified and selected by using its application identifier (AID). Commands such as selection command are formatted and transmitted in the form of application protocol data units (APDUs). Applets reply to each APDU command with a status word (SW) that indicates the result of the operation. An applet can optionally reply to an APDU command with other data.
Samrt card
A smart card size of credit card stores and processes information through the electronic circuits embedded in silicon in the plastic substrate of its body. An intelligent smart card contains a microprocessor and offers read, write, and calculating capability, like a small microcomputer. A smart card does not contain its own power supply, display, or keyboard. It interacts with a Card Acceptance Device (CAD) through using a communication interface, provided by a collection of eight electrical or optical contact points, as shown in fig,
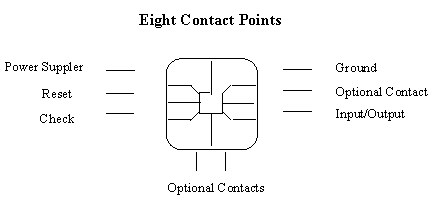
Java card
A Java Card is a smart card that is capable of running Java programs. The applications are devoloped in java using Java Card APIs. According to java card specification it supports only few primitive data types from Java programming language: byte, short, int and boolean. A byte is an 8-bit signed two’s complement number with a possible range of values between -128 to 127. A short is a 16-bit signed two’s complement number with a possible range of values between -32768 to 32767. Internally, Java Card technology represents the boolean type as a byte. The Java Card platform does not support threads because current smart card central processing units (CPUs) cannot support efficient multitasking. As a result, none of the thread keywords are supported. There is also no support for synchronized or volatile on the Java Card platform.
Virtual Machine
The Java Card Virtual Machine (VM) provides bytecode execution environment and Java language support, including exception handling. The Java Card Runtime Environment (JCRE) includes a virtual machine (VM) and core classes to support APDU routing, ISO communication protocols, and transaction-based processing. Java Card VM executes bytecode, manages classes and objects, enforces separation between applications (firewalls), and enables secure data sharing.
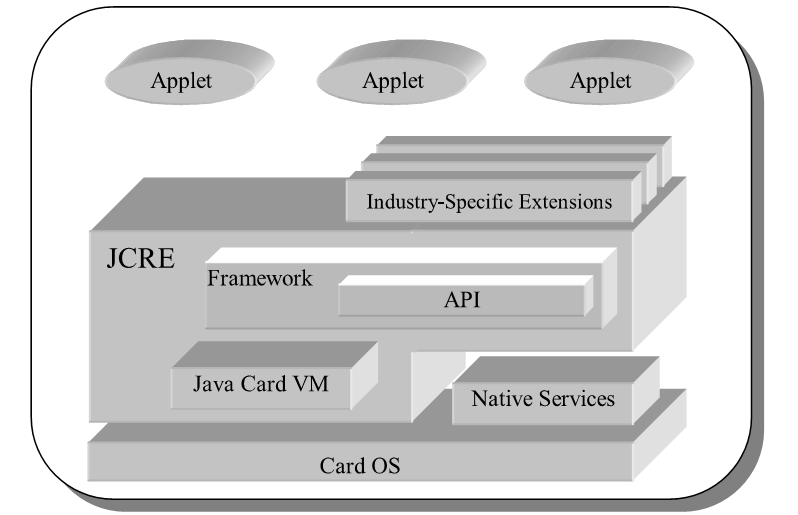
Application Protocol Data Units
When two computers communicate with each other, they exchange data packets, which are constructed following a set of protocols. Similarly, smart cards speak to the outside world using their own data packets -- called APDU (Application Protocol Data Units). Smart cards are reactive communicators—that is, they never initiate communications, they only respond to APDUs from the CAD (Card acceptance device). The communication model is command-response based—that is, the card receives a command APDU, performs the processing requested by the command, and returns a response APDU.
The following tables illustrate command and response APDU formats, respectively. APDU structure shown below is as described in ISO-7816.
CLA: Class byte. In many smart cards, this byte is used to identify an application.
INS: Instruction byte. This byte indicates the instruction code.
P1-P2: Parameter bytes. These provide further qualification to the APDU command.
Lc denotes the number of bytes in the data field of the command APDU.
Le denotes the maximum number of bytes expected in the data field of the following response APDU.
Java Card applet
Off-the-shelf Java technology development tools can be used to create and compile the source code for Java Card applets. For my project I used the tools,
1. NetBeans IDE 8.0.2
2. Java card development kit 3.0.2 Connected Edition
Once the Java software source code is compiled into a class file, a post-processed version of the applet, suitable for loading on Java technology smart cards is created using the Java Card Converter tool. An instance of applet is created and registered with the JCRE
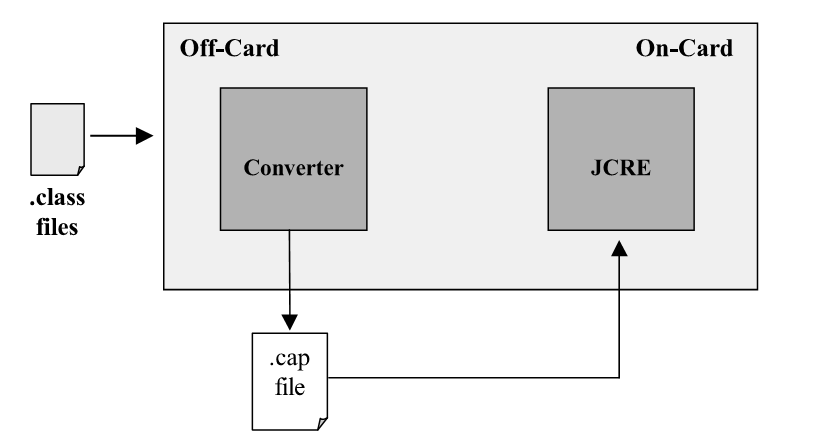
If you are new to Java Card and you really want to develop an application using java card, I suggest you having a look at Java Card Development Quick Start Guide and Writing a Java Card Applet. It will teach you how to start with java card and also it might be useful to follow this post and the code.
input
Applet receives APDU commands and data as an input and executes piece of code for the desired operation.
output
Applet outputs response APDU by setting the status words and data field (optional) in the APDU to indicate processing state of the card to termainal application.
Methods of javacard applet
1. install (byte[] bArray, short bOffset, byte bLength)The JCRE invokes this static method to create an instance of the Applet subclass.
2. select()
Invoked by JCRE to inform applet that it is being selected/not.
3. deselect ()
Invoked by the JCRE when a new select command is received to deselect currently selected applet and select another (or the same) applet.
4. process (APDU apdu)
Invoked by the JCRE to process an incoming APDU command.
5. register ()
This method is used by an applet to register this applet instance with JCRE and assign an ID to it.
6. register (byte[] bArray, short bOffset, byte bLength)
This method is used by an applet to register this applet instance with JCRE and to assign specified AID in the array bArray to an applet instance.
7. credit(APDU apdu)
This method is used to add amount to available balance. The amount is specified in the data field of the APDU.
8. debit(APDU apdu)
In this method, the APDU object contains a data field that specifies the amount to be debited from the balance.
9. getBalance(APDU apdu)
The method getBalance returns the Wallet's balance in the data field of the response APDU. Because the data field in the response APDU is optional, the applet must explicitly inform about the additional data.
Host Application
The Java tool chains are used to create and compile java card host application. A swing GUI has been designed to interact with the application. The GUI consists of buttons and text fields to set the commands and data in the APDU.Methods of the java card host application
1. establishConnectionToSimulator()This method establishes communication link with simulator activating port 9025 on localhost.
2. pwrUp()
In this method powerUp coomand is sent to power up the card
3. setTheAPDUCommands (byte[] cmnds)
This method sets the header of the APDU to be sent with commands (CLA, INS, P1, and P2) in APDU.
4. setTheDataLength (byte ln)
This method sets the (number of bytes present in the data field) Lc field of the APDU
5. setTheDataIn (byte[] data)
In this method, data fields of the APDU are set with data.
6. setExpctdByteLength (byte ln)
This method sets the number of bytes expected into the apdu.Le field.
7. exchangeTheAPDUWithSimulator()
This method exchanges the APDU with a card or simulator.
8. byte[] decodeDataOut()
In this method, the data from the applet in response APDU are decoded and returned the hex numbers to display on the GUI.
9. byte[] decodeStatusBytes()
This method decodes status words SW1 and SW2 of the response APDU and returns the status bytes to display on the GUI.
10. String atrToHex(byte atCode)
This method converts atr commands to hex numbers and returns the numbers in the form of strings.
11. pwrDown()
This method is used to power down the card.
12. closeConnection()
This method closes the socket connection.
The GUI
Graphical user interface has devided into two sections. On one section, the process of automatic teller machine can be simulated. In another section, the internal process can be visualized.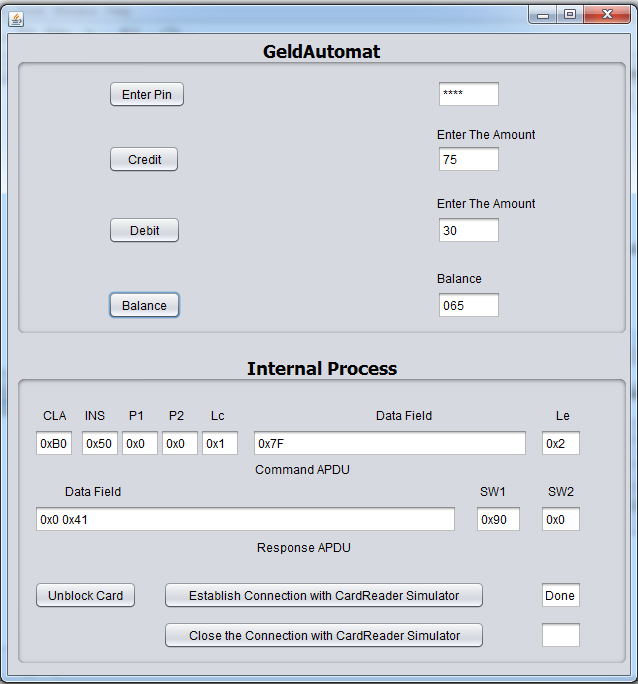